Friday, 07 Feb 2025
7 min read
Next.js Image Responsive Sizing: Media Query Guide
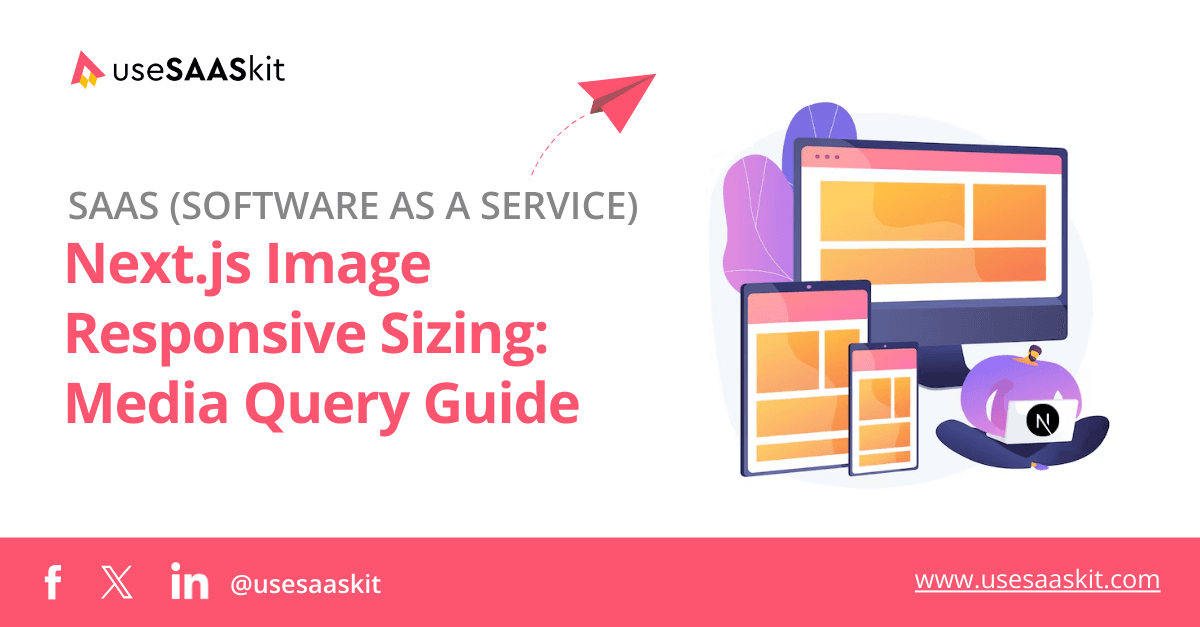
Responsive images are crucial for modern web development, as they adapt easily to various screen-size devices. The images will be the most vibrant, but not dragged down by any slow performance on the website. This optimization improves user experience, increases SEO rankings, and saves bandwidth. Now that mobile-friendly design is of great need, responsive images become a requirement for any site.
With its solid built-in functionalities and support for media queries, Next.js renders the management of responsive images easier. Next.js Image
component makes tasks like resizing, lazy loading, and optimization straightforward. When combined with media queries, you can deliver scalable, visual excellence with load times that please any device. This tutorial guides you through how to master the art of responsive image sizing using Next.js so that you can easily build web applications that are not only high-performing but also easy to use.
Table of Contents
- Why Responsive Images Matter in Modern Web Development
- Next.js Image Component: A Quick Overview
- Understanding Media Queries for Image Responsiveness
- Step-by-Step Guide to Implement Responsive Images in Next.js
- Best Practices for Responsive Images in Next.js
- Benefits of Using useSAASkit - Next.js SAAS Template
- Conclusion
- FAQs
Why Responsive Images Matter in Modern Web Development
Responsive images are important in today's web development landscape because they automatically adapt to different devices and screen resolutions. They make sure that images are sharp and proportional, whether the user is on a high-resolution desktop or a compact mobile screen. If images are not responsive, they can appear pixelated or distorted, which affects the overall design and user experience.
Responsiveness also helps reduce data usage in images by allowing the right size of image depending on the type of device; this reduces extra bandwidth consumption to make pages load faster and enable smoother browsing, thus improving the engagement of the users and ensuring better SEO ranks because search engines prefer faster, more efficient sites.
Managing responsive images is straightforward and efficient with Next.js. Tools like the Image
component allow developers to optimize their images automatically. Developers can thus save time, ensuring their web applications deliver great, high-performance experiences on any device. Therefore, it becomes an indispensable tool for modern web projects.
Next.js Image Component: A Quick Overview
The Next.js Image
component is a solid solution for automatic image optimization and ensures that everything works perfectly with the user. Its major advantages include the following:
- Automatic Resizing: This component adjusts image sizes automatically according to the device used by the user so that it will display optimally without overloading bandwidth. There is no need to have several different versions of the same image resized manually.
- Lazy Loading: Images are loaded only when they come into view, which greatly reduces the initial page load time and saves bandwidth. This is especially useful for image-heavy pages, where unnecessary preloading can slow down performance.
- Built-in Image Optimization: Next.js automatically compresses images to improve loading speed while maintaining visual quality. This ensures a balance between performance and aesthetics, which is essential for modern web applications.
- Placeholder Options: The component supports a blurred placeholder effect, which makes the page look more appealing during loading. Users find the page to be more polished and responsive, thus improving the overall user experience.
Using the Next.js Image
component, developers avoid the hassle of manually handling images and can, therefore, work on design and functionality. In addition to the technical simplification of image optimization, this component improves the performance and usability of web applications and is, thus, an important tool for creating high-quality websites and SaaS platforms.

Understanding Media Queries for Image Responsiveness
Media queries are CSS rules that change the style based on the device's properties, like screen size, resolution, or orientation. It is one of the main things that allow for responsive design, especially for images.
Media queries help you to make sure mobile devices get properly sized images and large, high-resolution images get served on the desktop. It's an optimization for user experience and performance.
Some of the main benefits of using media queries with responsive images include:
- Control Image Size: Use breakpoints to load the correct size for any image based on a given screen width
- Maintain Aspect Ratios: Ensure images maintain their dimensions across different devices
- Hide or Show: Hide or display certain elements based on the screen resolution, thereby providing a cleaner and more customized layout with less clutter.
The developer can create a visually consistent yet performance-oriented website that adjusts without any glitches on any device.
Step-by-Step Guide to Implement Responsive Images in Next.js
Using the Image
Component
The Next.js Image
component automatically optimizes images for responsiveness. Here's how to use it:
import Image from 'next/image';
const ResponsiveImage = () => (
<Image
src="/example.jpg"
alt="Example Image"
width={800}
height={600}
layout="responsive"
/>
);
Key properties:
layout="responsive"
: Ensures the image scales based on the container's width.width
andheight
: Define the aspect ratio for the image.
Optimizing with Breakpoints
Breakpoints are specific screen widths at which your design should adjust. Combine Next.js features with CSS media queries to manage breakpoints effectively:
/* styles.module.css */
.imageContainer {
width: 100%;
}
@media (min-width: 768px) {
.imageContainer {
width: 50%;
}
}
import styles from './styles.module.css';
const BreakpointImage = () => (
<div className={styles.imageContainer}>
<Image
src="/example.jpg"
alt="Example Image"
layout="responsive"
width={800}
height={600}
/>
</div>
);
This approach ensures images are displayed optimally on various screen sizes.
Combining CSS Modules and Media Queries
CSS Modules allow you to scope styles locally, making it easier to manage media queries for specific components. Here's an example:
/* styles.module.css */
.image {
border-radius: 10px;
}
@media (max-width: 600px) {
.image {
border-radius: 0;
}
}
import styles from './styles.module.css';
const StyledImage = () => (
<Image
className={styles.image}
src="/example.jpg"
alt="Styled Image"
layout="responsive"
width={800}
height={600}
/>
);
Best Practices for Responsive Images in Next.js
Master responsive images in Next.js with best practices such as defining clear breakpoints, optimizing image sizes, and using the Image component. Improve user experience, enhance SEO, and ensure your website looks great on every device.
- Define Clear Breakpoints: Plan device-specific breakpoints like 320px, 768px, and 1024px to maintain a consistent design across all screen sizes. This helps your images adapt seamlessly to varying device resolutions.
- Use Scalable Vector Graphics (SVGs): Use Scalable Vector Graphics (SVGs) for logos and icons. These lightweight files will provide sharp, crisp graphics whatever the size. They are ideal to be used in responsive designs.
- Optimize image sizes: Upload images at the highest resolution required, then let Next.js compress and resize them to deliver a good quality image with proper performance.
- Test Responsiveness Across Devices: Confirm whether images are rendered well by using the developer tools in your browsers and testing on real devices. This ensures that images render well on both small screens and large ones.
- Hybrid Server and Client-Side Rendering: Implement server-side rendering for those images that are critical for page loading. Any other visuals can utilize lazy loading to prevent bandwidth usage and improve performance.
By following these best practices, you can create visually appealing and highly optimized web apps with Next.js.

Benefits of Using useSAASkit - Next.js SAAS Template
Creating a responsive SaaS application can be a very complex job, but useSAASkit has made it much simpler and efficient. With pre-configured tools and a set of ready-made components, this template lets you focus more on the value addition in your platform rather than wasting your time on mundane repetitive tasks.
Key Benefits:
- Pre-configured Components: Ideal for launching websites quickly, the pre-configured components allow developers to reduce the added complexity and facilitate rapid launches.
- Optimized Performance: The template is built with performance and SEO in mind, ensuring your platform ranks well in search engines and delivers a seamless experience to users.
- Flexibility: Easily customize and adapt the template to suit your specific needs. Whether you're managing responsive images, building user-friendly dashboards, or scaling features, the useSAASkit template provides the versatility you need.
Leverage the usage of useSAASkit, hence less complexity during the development stage; concentrate more on high-performing responsive platforms aligned to the expectation of the modern user.
Conclusion
Responsive image sizing is a critical aspect of web development, as users want to see visuals that adapt naturally. Next.js does this without the effort by its powerful Image component. It automatically optimizes images, further enhanced when combined with media queries. It is a key feature for e-commerce platforms and content-heavy websites because it contributes to making user experiences better and enhancing performance.
For an easier development process, the use of useSAASkit is a great choice. It offers pre-configured components and tools that help you build scalable and efficient platforms quickly while handling responsive images effortlessly.
FAQs
What is the purpose of the Next.js Image component?
The Next.js Image component is a utility for optimizing images automatically and also includes lazy loading, resizing, and more.
How do media queries help in making images responsive?
Media queries let the developers declare various styles or sizes of an image according to device properties, like screen width or resolution.
Why are responsive images crucial for SEO?
Responsive images improve page-load speed, an important factor for SEO, and enhance the user experience by serving optimized images.
Can I use Next.js Image with external image URLs?
Yes, you can configure external domains in the next.config.js file to use external image URLs with the Next.js Image component.